Next.js overview in 1000 words
Next.js is one of many static site generators. But it has one feature that stands out from the competition. In this brief overview, I'll try to describe it.
Last updated: November 28, 2023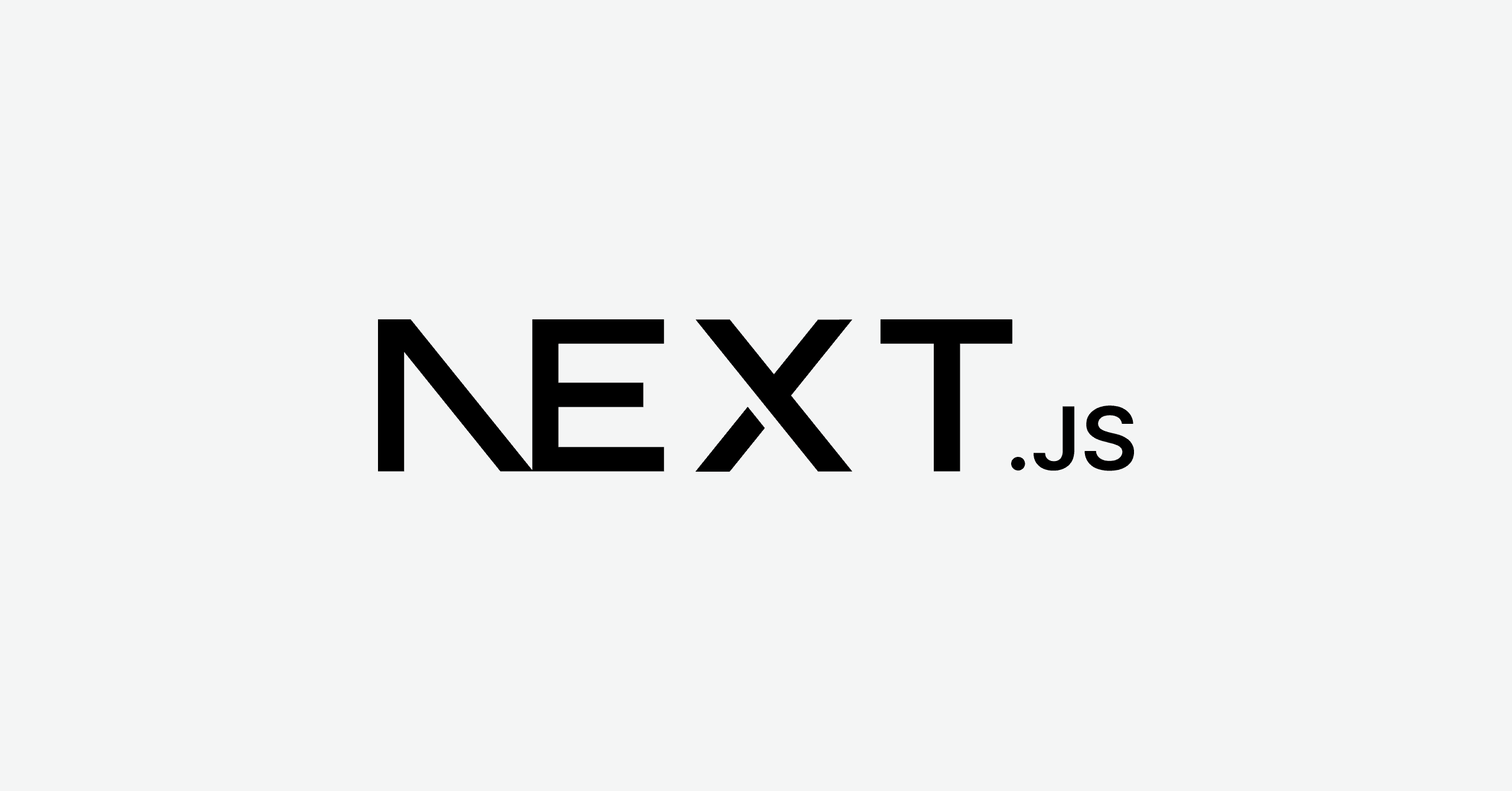
"Every day, a new JavaScript framework is born," people say. The sentence is exaggerated and funny, but there is some truth. In the rapidly changing world of JavaScript development, it's easy to pick up a new, shiny tool and ditch the old one. However, it often turns out that the new framework is not the best for our use case. Keeping that in mind, I promised myself I would not hop on any hype train. But with the new release of Next.js and almost 100 000 stars on GitHub, the hype train is REAL. Still, instead of blindly boarding, I'll try to make a brief overview of Next.js. And I'll attempt to make it in 1000 words.
Next.js is a React framework
First, let's clear some misconceptions I saw. Next.js is not an alternative to frameworks like Vue, Angular, or React. It's more of an alternative to static site generators like Gatsby or Hugo (it's not 100% accurate, but we'll get to that). You don't use React or Next.js. Of course, you can choose between them, but you use React either way. Next builds on top of React. So, we can say it's a framework2 or meta-framework.
If you want to see static site generators in context, I wrote a post about the whole Jamstack architecture.
Why use meta-framework?
"Why the hell do I need to use meta-framework? Is React not enough?" you may ask. And that depends. It depends on the thing you want to build. If you want to create a simple SPA, React is probably enough. But websites in production need to solve problems with routing, SEO, image optimization, and more. React doesn't offer that, and Next.js does. It helps solve these commonly occurring problems. The home page of Next states: "The React Framework for Production," and it concisely explains what it is.
Rendering strategies
There is another problem with SPAs - client-side rendering. The user experience they provide is similar to native apps, which is excellent, but they have some downsides. The biggest two are:
- SEO problems. When a server sends React SPA, it serves a blank page with a JavaScript bundle that builds the website on the client. So, there are no meta tags like a title, description, or data for Twitter cards. The content is not reliably visible to search engines like Google, Twitter, or Facebook. Googlebot can crawl websites based on JavaScript, but you shouldn't rely on that if indexing is a priority for you.
- Slower First Contentful Paint. FCP is one of the metrics used to measure web page performance. It's the time required to display the first DOM element. And because with SPAs, we send a blank page first, the results are usually worse than in the server-side rendering.
Server-Side Rendering
SSR is not a new strategy. If you used PHP or .NET, you have experience with it. SSR with JavaScript works similarly but with Node.js as the runtime environment. As the name suggests, a server is responsible for building a page. The server generates each page at the request. It eliminates the problems mentioned above, but we lose native-like abilities. Navigating between pages triggers browser refreshes. The user experience is worse.
Static Site Generation
SSG moves the render process from the server into the build process. A static site generator like Next will request a list of all the pages and render them with all the necessary files. Then you can take those static files and deploy them to Netlify or another CDN. This strategy combines the advantages of previous methods: we keep SPA interactive capabilities, and we have content for web crawlers. Both users and machines are happy. This strategy is even faster than server-side rendering because of the content pre-rendering. "Ok, so we have a winner here. Can we go home now?" Not so fast. We need to remember the build phase. Dynamic data can't be pre-built, and the build process can be lengthy for websites with thousands of pages.
Next.js rendering strategies
Why babble so much about rendering methods in the Next.js post? Because I think that's the main selling point of Next.js. Now (from the 9.3 version, to be precise), it offers all of them. There are different data fetching functions.
useSWR
The team behind Next.js has created a custom React hook for data fetching. It is a recommended way to fetch data on the client side.
import useSWR from 'swr'
const fetcher = (...args) => fetch(...args).then((res) => res.json())
function Profile() {
const { data, error } = useSWR('/api/profile-data', fetcher)
}
getServerSideProps
Exporting getServerSideProps
from a page allows rendering the page on each request. The page will use the data returned from this function.
export async function getServerSideProps(context) {
return {
props: {}
}
}
getStaticProps
You can use static site generation with the getStaticProps
function. Next.js uses returned data to pre-render a page at build time. This function works with getStaticPaths
to generate paths dynamically from the delivered content.
export async function getStaticProps(context) {
return {
props: {}
}
}
Incremental Static Regeneration
The strategy is specific to Next.js. It attempts to solve static site generation issues. It allows you to update static pages after you've built your website. So you no longer need to rebuild the entire thing. To use it, you need to add the revalidate
prop to getStaticProps
.
export async function getStaticProps(context) {
return {
props: {},
revalidate: 10
}
}
The best thing is you can pick a rendering strategy on a per-route basis. You can statically generate one part of your website and server-side render the other. And that's an advantage over other static site generators like Gatsby, which mainly focuses on static generation. Next.js covers more use cases.
Other features of Next.js
Besides multiple data fetching methods, Next.js offers solutions for routing (and transitions between routes with next/link
), redirects, and image/font optimization, including meta tags or other scripts. It supports Typescript out of the box and multiple options for styling and testing (Jest and Cypress). There is also Next.js CLI that allows managing your app. This post was supposed to be a brief overview of Next.js, and I'm closing to 1000 words, so I'll end here. But the framework seems interesting to me, and I may learn it. I feel switching between static generators won't be so hard. I may add more detailed posts about its different features.
Next.js 13 introduced the app router. Besides switching to a new routing strategy, this version introduced more changes like server components. My post refers to the previous pages router. But all the information in this post is still relevant. You can still use the pages router. Rendering strategies didn't change, but their implementation may differ. See the Next.js docs for more details.